Listing POIs - All
This sample shows how to get detailed information about all POIs in a venue. This may be useful when you want to compose venue wide data.
Organizing your code
The Getting Started section details various SDK installation options depending on your requirements. The sample code below is based on a base use case of a plain html page and the ES6 module format.
Get all POIS
Update your code to request all POIs and log some data for each POI returned:
<!DOCTYPE html>
<html lang="en">
<head>
<script type="module">
import LMInit from 'https://maps.locuslabs.com/sdk/LocusMapsSDK.js'
const config = {
venueId: 'lax',
accountId: 'A11F4Y6SZRXH4X',
headless: true
}
window.LMInit = LMInit
LMInit.setLogging(true)
LMInit.newMap(null, config)
.then(m => {
window.map = m;
console.log("ready");
m.getAllPOIs().then(pois => {
for (const [poiID, poi] of Object.entries(pois)) {
console.log("ID: ", poiID);
console.log("Name: ", poi.name);
console.log("Category: ", poi.category);
console.log("Position: ", poi.position);
}
});
})
.catch(e => console.error('Error initializing map: ', e))
</script>
</head>
</html>
Try it out
See a live preview (inspect the logs).
Below is an extract of the data that will be returned in the console If you run the code above:
ID: – "804"
Name: – "Police"
Category: – "services.police"
Position: – {floorId: "lax-south-departures", latitude: 33.94202, longitude: -118.406928, …}
ID: – "808"
Name: – "Japan Airlines Check-In"
...
Other POI Data
In addition the fields highlighted above, the POI object contains many other fields like "links", "images", "phone" and more. Some fields may not be returned for all POIs. The screenshot below shows all fields returned for a typical POI:
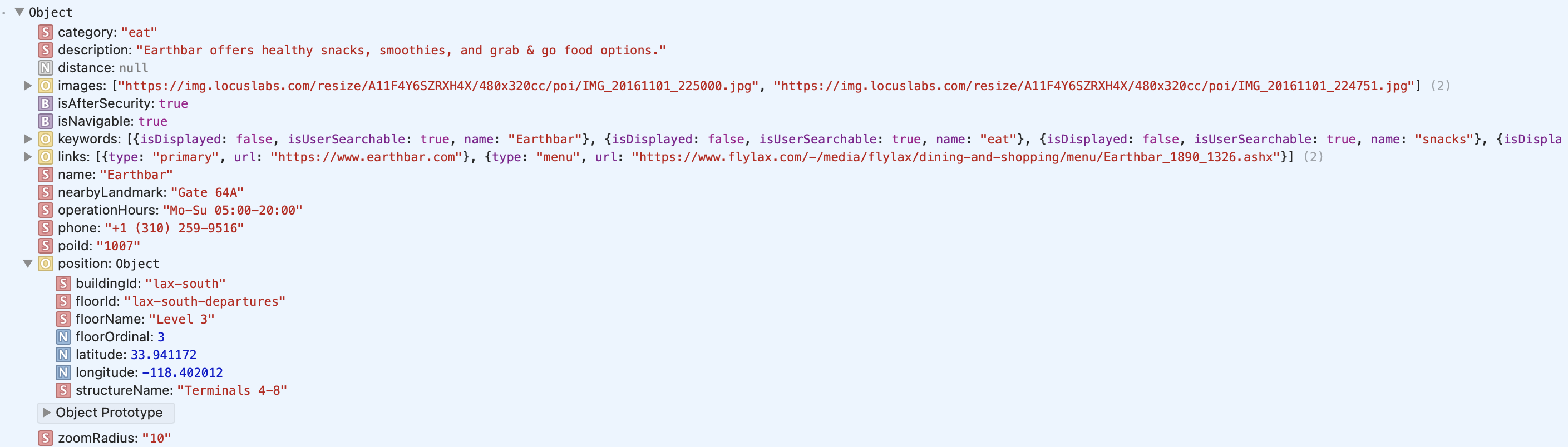
Updated over 1 year ago