Auto Display Searches
This sample shows how to trigger a programmatic search that will automatically display the results on the map.
Organizing your code
The Getting Started section details various SDK installation options depending on your requirements. The sample code below is based on a base use case of a plain html page and the ES6 module format.
Automatically display search results
The code below shows how to request the map to automatically display the results of a programmatic search. This is achieved using the "showSearch" command which will fill the search box with the passed in term and highlight the results on the map:
<!DOCTYPE html>
<html lang="en">
<head>
<script type="module">
import LMInit from 'https://maps.locuslabs.com/sdk/LocusMapsSDK.js'
const config = {
venueId: 'lax',
accountId: 'A11F4Y6SZRXH4X',
headless: false
}
window.LMInit = LMInit
LMInit.setLogging(true)
LMInit.newMap('.mymap', config)
.then(m => {
window.map = m;
map.showSearch("restaurant");
})
.catch(e => console.error('Error initializing map: ', e))
</script>
<style>
html, body, .mymap {
height: 100%; margin: 0; padding: 0;
}
</style>
</head>
<body>
<div class="mymap" style="height: 100%;"></div>
</body>
</html>
Try it out
See a live preview here. After the search results have been returned, the map will appear similar to the following image:
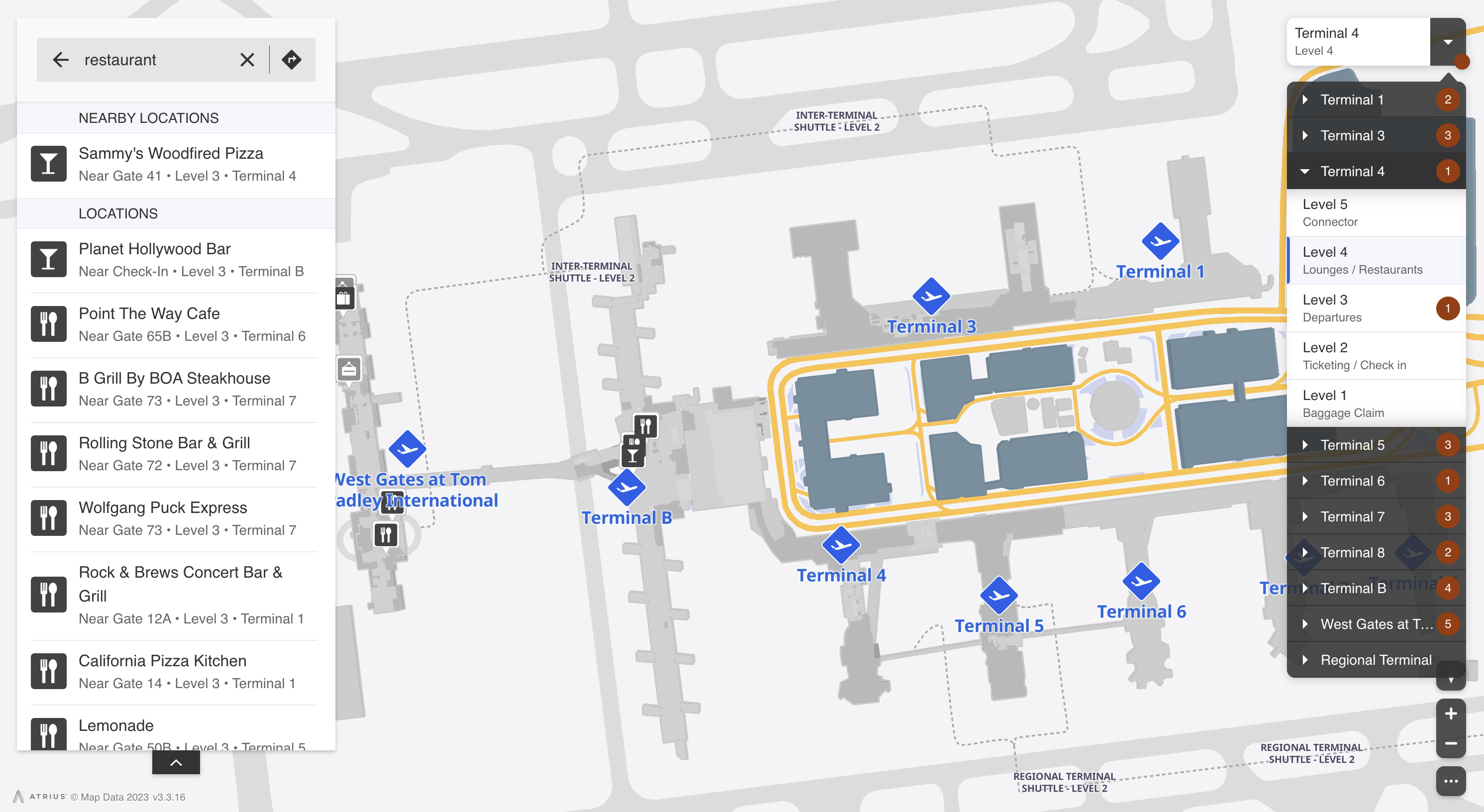
Clearing Search Results
Use the "resetSearch" command to clear the search bar and highlighted results from the map.
Updated about 1 year ago