Show Search Results
This sample shows how to launch the map showing the results of a specific search. There are 2 ways to do so:
- Specify the search term in the launch config
- Include the search term directly in the URL
Organizing your code
The Getting Started section details various SDK installation options depending on your requirements. The sample code below is based on a base use case of a plain html page and the ES6 module format.
Specify the search term in the launch config
Update the base code to include the "deepLinksParms" key in the launch config. Specifically, we will ask the SDK to show the results of a search for "restaurant" as shown below:
<html lang="en">
<head>
<script type="module">
import LMInit from 'https://maps.locuslabs.com/sdk/LocusMapsSDK.js'
const config = {
venueId: 'lax',
accountId: 'A11F4Y6SZRXH4X',
headless: false,
deepLinkParms: {search: "restaurant"}
}
window.LMInit = LMInit
LMInit.setLogging(true)
LMInit.newMap('.mymap', config)
.then(m => { window.map = m; })
.catch(e => console.error('Error initializing map: ', e))
</script>
<style>
html, body, .mymap {
height: 100%; margin: 0; padding: 0;
}
</style>
</head>
<body>
<div class="mymap" style="height: 100%;"></div>
</body>
</html>
Try it out
See a live preview or visit the fiddle (note - the fiddle may only display the mobile version due to size constraints).
After loading, the map will show the search results and appear similar to the image below:
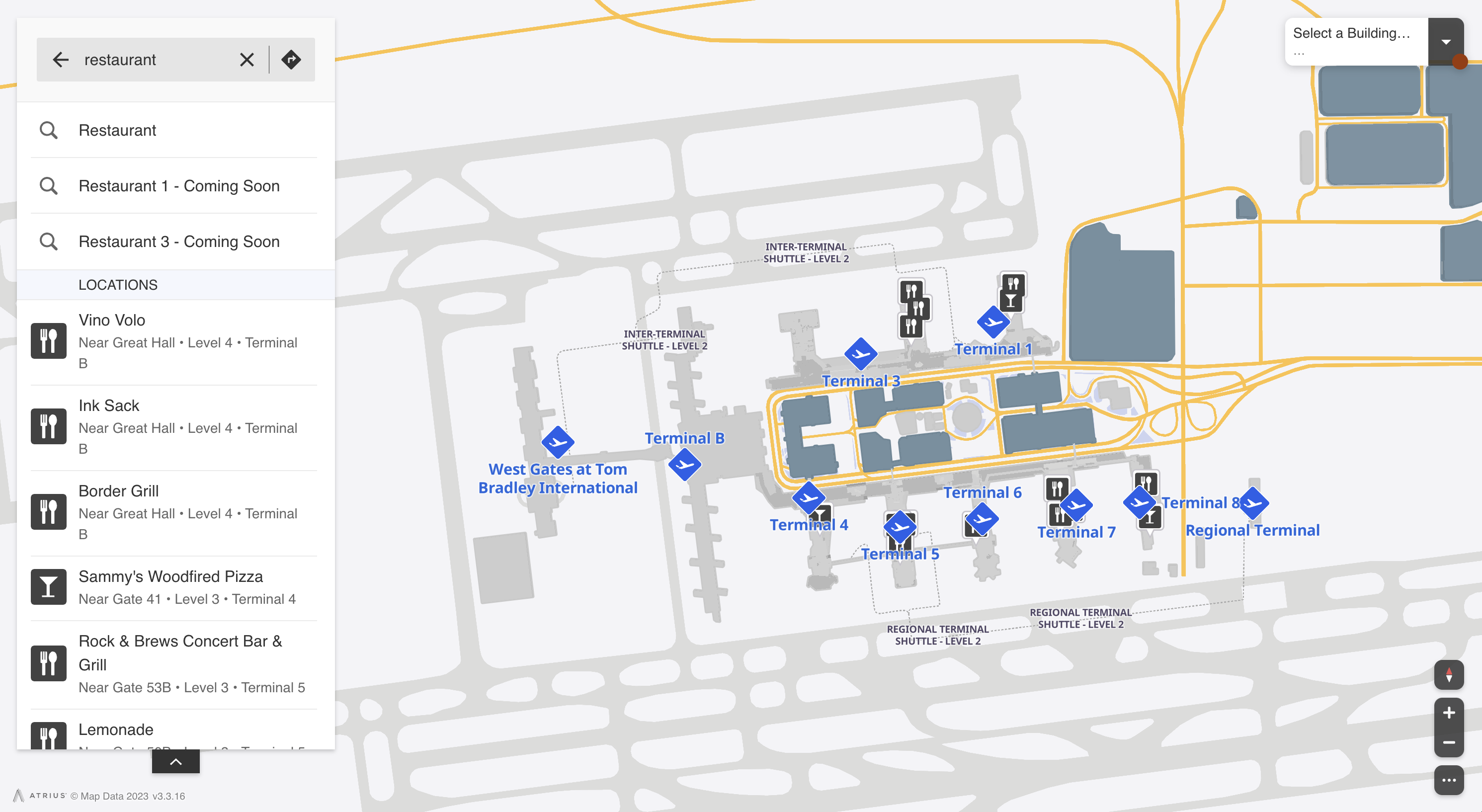
Specify the search term in the URL
You can also show the search results at launch simply by adding the search term to the map URL as follows:
<your_map_url>?search=<search_term>
For the above to work, you need to specify in your launch config that url deep links are accepted by adding the "supportURLDeepLinks" key as shown below:
<html lang="en">
<head>
<script type="module">
import LMInit from 'https://apps.locuslabs.com/sdk/dist/current/LocusMapsSDK.js'
const config = {
venueId: 'lax',
accountId: 'A11F4Y6SZRXH4X',
headless: false,
supportURLDeepLinks: true
}
window.LMInit = LMInit
LMInit.setLogging(true)
LMInit.newMap('.mymap', config, "https://apps.locuslabs.com/sdk/dist/current/")
.then(m => { window.map = m })
.catch(e => console.error('Error initializing map: ', e))
</script>
<style>
html, body, .mymap {
height: 100%; margin: 0; padding: 0;
}
</style>
</head>
<body>
<div class="mymap" style="height: 100%;"></div>
</body>
</html>
Try it out
Click here to see the map open showing the search results using only a URL param.
Updated 2 months ago