Showing a POI
This sample shows how to programmatically show a POI overlay at any time or directly at launch.
Organizing your code
The Getting Started section details various SDK installation options depending on your requirements. The sample code below is based on a base use case of a plain html page and the ES6 module format.
Add the showPOI command
The code below shows how show the the info overlay for any POI by passing in its id to the "showPOI" command. This command will also move and zoom the map to the POI and highlight it. The code below shows the POI for Earthbar near Gate 64:
<!DOCTYPE html>
<html lang="en">
<head>
<script type="module">
import LMInit from 'https://maps.locuslabs.com/sdk/LocusMapsSDK.js'
const config = {
venueId: 'lax',
accountId: 'A11F4Y6SZRXH4X',
headless: false
}
window.LMInit = LMInit
LMInit.setLogging(true)
LMInit.newMap('.mymap', config)
.then(m => {
window.map = m;
// Show the Earthbar POI overlay and move the map to it
map.showPOI(1007);
})
.catch(e => console.error('Error initializing map: ', e))
</script>
<style>
html, body, .mymap {
height: 100%; margin: 0; padding: 0;
}
</style>
</head>
<body>
<div class="mymap" style="height: 100%;"></div>
</body>
</html>
Try it out
See a live preview here. After the POI shows, the map will appear similar to the following image:
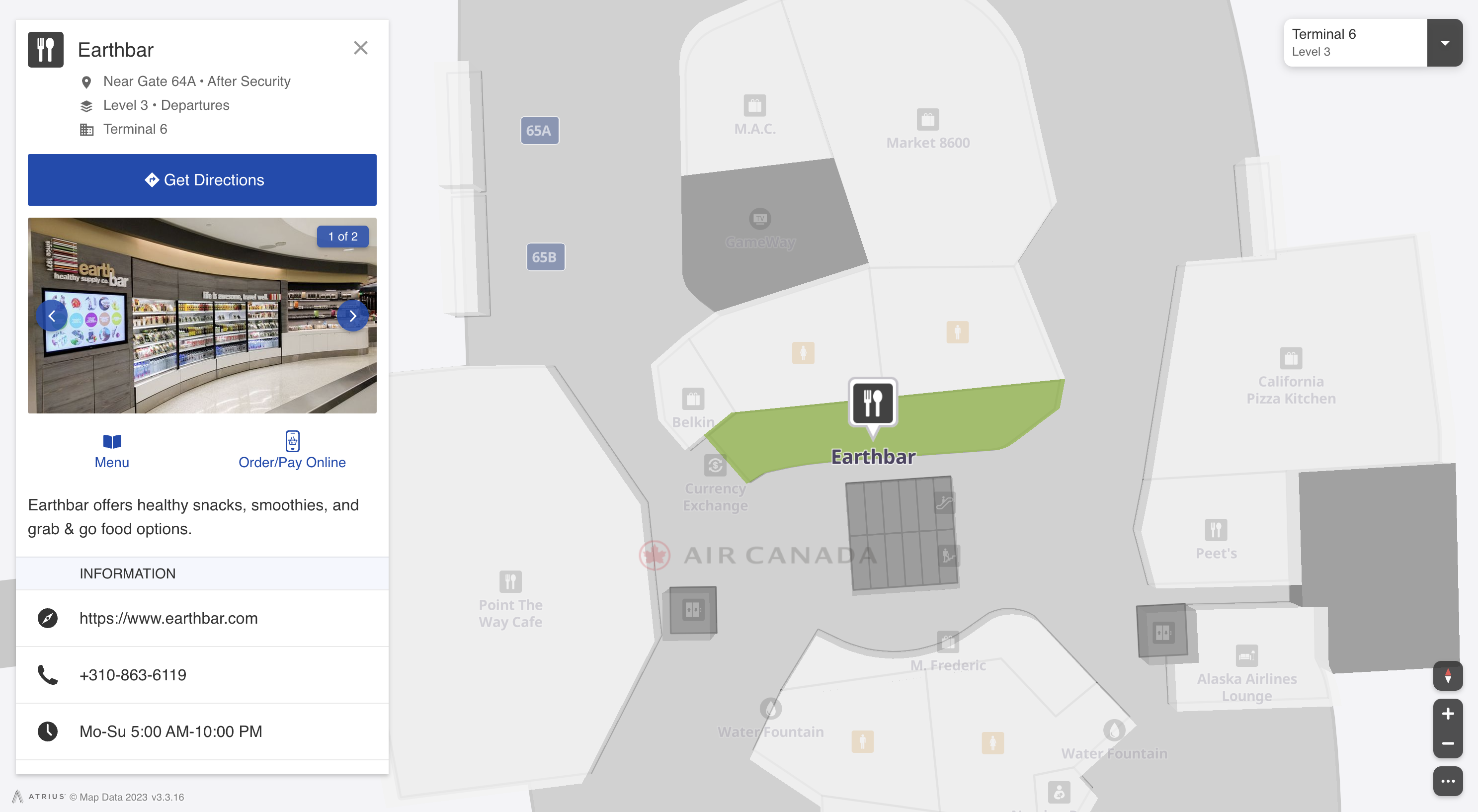
Updated almost 2 years ago