Map Markers
This sample shows how to add a custom marker to any point on the map and also how to remove it.
Organizing your code
The Getting Started section details various SDK installation options depending on your requirements. The sample code below is based on a base use case of a plain html page and the ES6 module format.
Add the marker
The code below shows how to add a custom marker close to Gate 64 of Terminal 6 in LAX (note that the last param is optional and if omitted, a standard marker will be shown):
<!DOCTYPE html>
<html lang="en">
<head>
<script type="module">
import LMInit from 'https://maps.locuslabs.com/sdk/LocusMapsSDK.js'
const config = {
venueId: 'lax',
accountId: 'A11F4Y6SZRXH4X',
headless: false
}
window.LMInit = LMInit
LMInit.setLogging(true)
LMInit.newMap('.mymap', config)
.then(m => {
window.map = m;
map.drawMarker("Marker1", {lat:33.9414, lng:-118.4019, ord:3}, "https://locuslabs-documentation-samples.s3.us-east-2.amazonaws.com/red_pin.svg"); })
.catch(e => console.error('Error initializing map: ', e))
</script>
<style>
html, body, .mymap {
height: 100%; margin: 0; padding: 0;
}
</style>
</head>
<body>
<div class="mymap" style="height: 100%;"></div>
</body>
</html>
Showing a marker at a POI
The code above shows how to add a marker to a specific point on the map using coordinates, but a marker can also be placed on a POI by passing in the POI ID in stead of the coordinates to the "drawMarker" command e.g. {poiId: 1007}
Removing a Marker
To remove a marker previous added, simply use the "hideMarker" command, passing in the name used when adding the marker. For the same code above, the marker can be used by issuing the command map.hideMarker("Marker1")
.
Marker Image Format
Marker images can be in png, jpg or svg format. SVG is recommended at a base size of 30-50px.
Try it out
See a live preview here. The map will appear similar to the following image, with the red marker clearly visible:
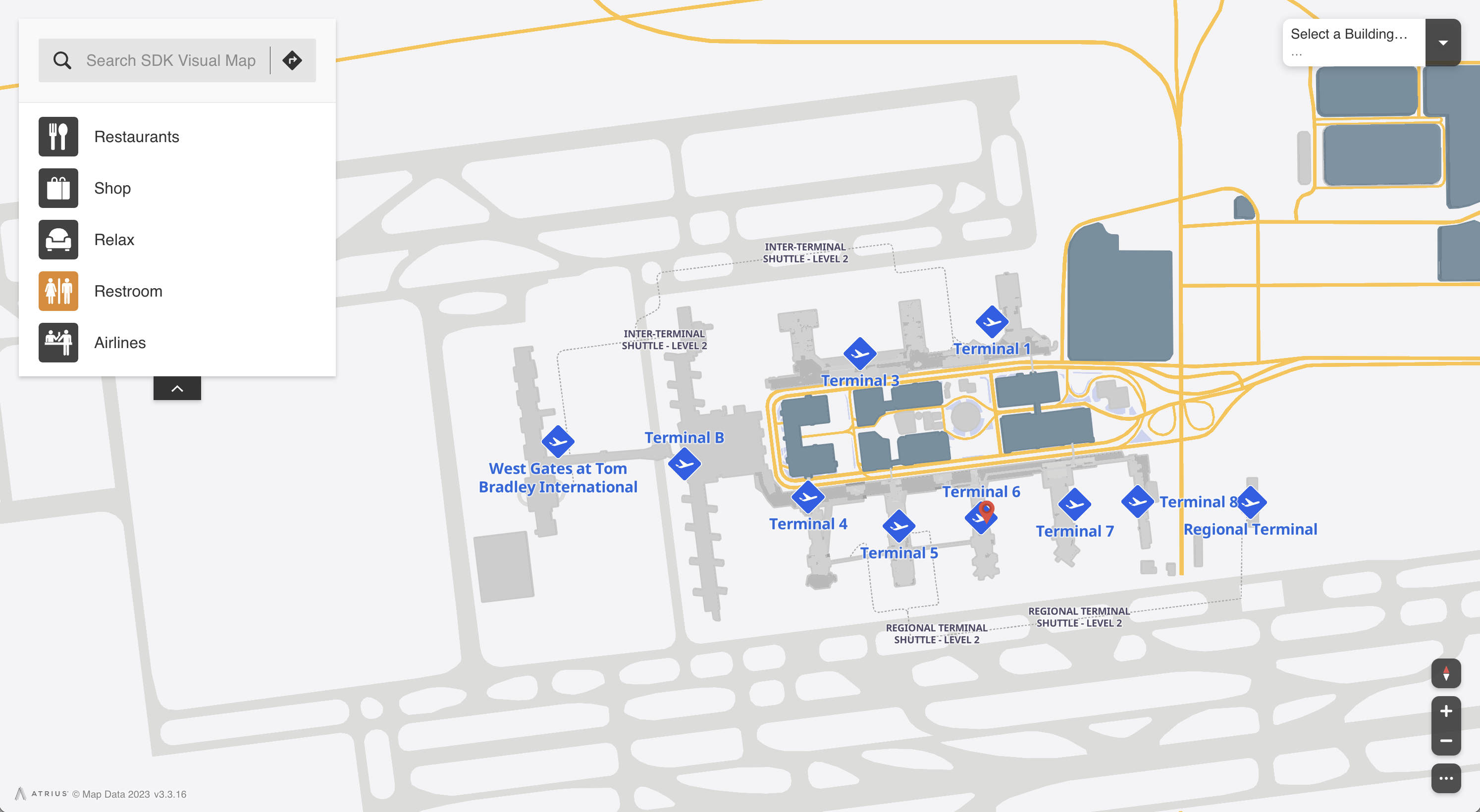
Updated over 1 year ago